Merge branch 'feature/freeMailAct' into 'release/6.0'
免邮券领取页 See merge request !740
Showing
24 changed files
with
595 additions
and
0 deletions
apps/activity/controllers/free-mail.js
0 → 100644
1 | +'use strict'; | ||
2 | + | ||
3 | +const freeMailModel = require('../models/free-mail'); | ||
4 | + | ||
5 | +exports.freeMailIndex = (req, res) => { | ||
6 | + | ||
7 | + res.render('free-mail/index', { | ||
8 | + page: 'free-mail-index', | ||
9 | + localCss: true | ||
10 | + }); | ||
11 | +}; | ||
12 | + | ||
13 | +exports.freeMailList = (req, res, next) => { | ||
14 | + let uid = req.user.uid; | ||
15 | + | ||
16 | + req.ctx(freeMailModel).freeMail(uid).then(result => { | ||
17 | + res.render('free-mail/list', { | ||
18 | + page: 'free-mail-list', | ||
19 | + localCss: true, | ||
20 | + listData: result | ||
21 | + }); | ||
22 | + }).catch(next); | ||
23 | +}; | ||
24 | + | ||
25 | +exports.receiveVerify = (req, res, next) => { | ||
26 | + let uid = req.user.uid, | ||
27 | + verify = true; | ||
28 | + | ||
29 | + req.ctx(freeMailModel).freeMail(uid, verify).then(result => { | ||
30 | + res.json(result); | ||
31 | + }).catch(next); | ||
32 | +}; | ||
33 | + | ||
34 | +exports.receiveCoupon = (req, res, next) => { | ||
35 | + let uid = req.user.uid; | ||
36 | + | ||
37 | + req.ctx(freeMailModel).receiveCoupon(uid).then(result => { | ||
38 | + res.json(result); | ||
39 | + }).catch(next); | ||
40 | +}; |
apps/activity/models/free-mail.js
0 → 100644
1 | +const api = global.yoho.API; | ||
2 | +const _ = require('lodash'); | ||
3 | +const moment = require('moment'); | ||
4 | + | ||
5 | +module.exports = class extends global.yoho.BaseModel { | ||
6 | + constructor(ctx) { | ||
7 | + super(ctx); | ||
8 | + } | ||
9 | + | ||
10 | + /** | ||
11 | + * 领取验证以及领卷列表 | ||
12 | + */ | ||
13 | + freeMail(uid, verify) { | ||
14 | + return api.get('', { | ||
15 | + method: 'app.coupons.queryFreePostCoupons', | ||
16 | + uid: uid | ||
17 | + }).then((result) => { | ||
18 | + if (verify) { | ||
19 | + return result; | ||
20 | + } else { | ||
21 | + if (result && result.code === 200) { | ||
22 | + | ||
23 | + _.forEach(result.data.freePostCoupons, function(data) { | ||
24 | + data.startTime = moment.unix(data.startTime).format('YYYY.M.D'); | ||
25 | + data.endTime = moment.unix(data.endTime).format('YYYY.M.D'); | ||
26 | + }); | ||
27 | + | ||
28 | + return result.data; | ||
29 | + } else { | ||
30 | + return {}; | ||
31 | + } | ||
32 | + } | ||
33 | + }); | ||
34 | + } | ||
35 | + | ||
36 | + receiveCoupon(uid) { | ||
37 | + return api.get('', { | ||
38 | + method: 'app.coupons.getFreePostCoupons', | ||
39 | + uid: uid | ||
40 | + }).then((result) => { | ||
41 | + return result; | ||
42 | + }); | ||
43 | + } | ||
44 | +}; |
@@ -53,6 +53,7 @@ const userRecommend = require(`${cRoot}/user-recommend`); | @@ -53,6 +53,7 @@ const userRecommend = require(`${cRoot}/user-recommend`); | ||
53 | 53 | ||
54 | const expand = require(`${cRoot}/expand-new`); | 54 | const expand = require(`${cRoot}/expand-new`); |
55 | 55 | ||
56 | +const freeMail = require(`${cRoot}/free-mail`); | ||
56 | 57 | ||
57 | // routers | 58 | // routers |
58 | 59 | ||
@@ -267,4 +268,9 @@ router.get('/my-reward', auth, expand.myReward); // 拓展新客 我的奖励页 | @@ -267,4 +268,9 @@ router.get('/my-reward', auth, expand.myReward); // 拓展新客 我的奖励页 | ||
267 | router.get('/reward-list', expand.rewardList); // 拓展新客 我的奖励列表 | 268 | router.get('/reward-list', expand.rewardList); // 拓展新客 我的奖励列表 |
268 | router.get('/reward-detail', auth, expand.rewardDetail); // 拓展新客 我的奖励详情页 | 269 | router.get('/reward-detail', auth, expand.rewardDetail); // 拓展新客 我的奖励详情页 |
269 | 270 | ||
271 | +router.get('/free-mail/index', auth, freeMail.freeMailIndex); // 免邮券主页 | ||
272 | +router.get('/free-mail/list', auth, freeMail.freeMailList); // 免邮券列表页 | ||
273 | +router.get('/free-mail/verify', auth, freeMail.receiveVerify); // 免邮券领取验证 | ||
274 | +router.get('/free-mail/verifyCoupon', auth, freeMail.receiveCoupon); // 免邮券领取 | ||
275 | + | ||
270 | module.exports = router; | 276 | module.exports = router; |
1 | +<div class="free-mail-index-page yoho-page"> | ||
2 | + <div class="top-banner"> | ||
3 | + <span class="receive-btn"></span> | ||
4 | + </div> | ||
5 | + <div class="instructions"> | ||
6 | + <div class="instructions-detail"> | ||
7 | + <div class="title"></div> | ||
8 | + <div class="detail-item time"> | ||
9 | + <div class="item-left"> | ||
10 | + <span class="icon"></span> | ||
11 | + <span class="item-title">领取时间</span> | ||
12 | + </div> | ||
13 | + <div class="item-right"> | ||
14 | + <span><b class="color">每月均可领取,</b>领取之日起至当月月底有效,过期不补偿,领取后请尽快使用</span> | ||
15 | + </div> | ||
16 | + </div> | ||
17 | + | ||
18 | + <div class="detail-item status"> | ||
19 | + <div class="item-left"> | ||
20 | + <span class="icon"></span> | ||
21 | + <span class="item-title">领取资格</span> | ||
22 | + </div> | ||
23 | + <div class="item-right"> | ||
24 | + <span>您在领取时,<b class="color">必须是有货VIP金卡、白金卡用户。</b><a href="//m.yohobuy.com/home/grade">(了解您当前的VIP级别)</a> 金卡会员3张运费券,白金会员5张运费券。 | ||
25 | + </span> | ||
26 | + </div> | ||
27 | + </div> | ||
28 | + | ||
29 | + <div class="detail-item tip"> | ||
30 | + <div class="item-left"> | ||
31 | + <span class="icon"></span> | ||
32 | + <span class="item-title">温馨提示</span> | ||
33 | + </div> | ||
34 | + <div class="item-right"> | ||
35 | + <span> | ||
36 | + 运费券下单时账户自动扣减相应金额运费,<b class="color">可与优惠券叠加使用,</b>如后期产生退货,运费券不退回。 | ||
37 | + </span> | ||
38 | + </div> | ||
39 | + </div> | ||
40 | + </div> | ||
41 | + </div> | ||
42 | + | ||
43 | + <div class="pop-up"> | ||
44 | + </div> | ||
45 | +</div> |
1 | +<div class="free-mail-list-page yoho-page"> | ||
2 | + {{# listData}} | ||
3 | + <div class="top-banner"> | ||
4 | + <span class="coupon-name">{{name}}</span> | ||
5 | + </div> | ||
6 | + <div class="coupon-list"> | ||
7 | + {{# freePostCoupons}} | ||
8 | + <div class="coupon-item"> | ||
9 | + <div class="item-left"> | ||
10 | + <span class="name">{{couponName}}</span> | ||
11 | + <span class="time">{{startTime}}-{{endTime}}</span> | ||
12 | + </div> | ||
13 | + <div class="item-right"> | ||
14 | + <span class="amount">{{amount}}</span> | ||
15 | + </div> | ||
16 | + </div> | ||
17 | + {{/ freePostCoupons}} | ||
18 | + </div> | ||
19 | + <div class="receive-btn"></div> | ||
20 | + {{/ listData}} | ||
21 | +</div> |
public/img/activity/free-mail/banner.png
0 → 100644

229 KB
public/img/activity/free-mail/coupon.png
0 → 100644

12.9 KB
public/img/activity/free-mail/failure.png
0 → 100644

6.1 KB

153 KB
public/img/activity/free-mail/list-btn.png
0 → 100644

17.2 KB
public/img/activity/free-mail/status.png
0 → 100644

4.24 KB
public/img/activity/free-mail/time.png
0 → 100644

4.45 KB
public/img/activity/free-mail/tip.png
0 → 100644

3.91 KB
public/img/activity/free-mail/title.png
0 → 100644
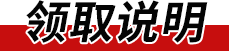
8.88 KB
1 | +'use strict'; | ||
2 | +const yoho = require('yoho-app'); | ||
3 | + | ||
4 | +import { | ||
5 | + Controller | ||
6 | +} from 'yoho-mvc'; | ||
7 | + | ||
8 | +import { | ||
9 | + IndexView | ||
10 | +} from './view'; | ||
11 | + | ||
12 | +import { | ||
13 | + FreeMail | ||
14 | +} from './model'; | ||
15 | + | ||
16 | +class FreeMailIndexController extends Controller { | ||
17 | + constructor() { | ||
18 | + super(); | ||
19 | + this.receiveView = new IndexView(); | ||
20 | + this.freeMailModel = new FreeMail(); | ||
21 | + this.receiveView.on('goReceive', this.goReceive.bind(this)); | ||
22 | + } | ||
23 | + | ||
24 | + goReceive() { | ||
25 | + this.freeMailModel.receiveVerify().then(result => { | ||
26 | + if (result.code === 200) { | ||
27 | + if (yoho.isApp) { | ||
28 | + yoho.goH5('http://m.yohobuy.com/activity/free-mail/list', JSON.stringify({ | ||
29 | + action: 'go.h5', | ||
30 | + params: { | ||
31 | + islogin: 'N', | ||
32 | + url: 'http://m.yohobuy.com/activity/free-mail/list' | ||
33 | + } | ||
34 | + })); | ||
35 | + } else { | ||
36 | + location.href = '//m.yohobuy.com/activity/free-mail/list'; | ||
37 | + } | ||
38 | + } else if (result.code === 419) { | ||
39 | + $('.pop-up').html('<div class="details"><span></span>' + result.message + '</div>').show(); | ||
40 | + } else if (result.code === 418) { | ||
41 | + $('.pop-up').html('<div class="big">本月已领取过<br>下月再来哦~</div>').show(); | ||
42 | + } else { | ||
43 | + $('.pop-up').html(result.message).show(); | ||
44 | + } | ||
45 | + | ||
46 | + this.receiveView.popHide(); | ||
47 | + }); | ||
48 | + } | ||
49 | +} | ||
50 | + | ||
51 | +module.exports = FreeMailIndexController; |
public/js/activity/free-mail-index/index.js
0 → 100644
public/js/activity/free-mail-index/model.js
0 → 100644
public/js/activity/free-mail-index/view.js
0 → 100644
1 | +import { | ||
2 | + View | ||
3 | +} from 'yoho-mvc'; | ||
4 | + | ||
5 | +class IndexView extends View { | ||
6 | + constructor() { | ||
7 | + super('.top-banner'); | ||
8 | + | ||
9 | + this.receiveBtn = $('.receive-btn'); | ||
10 | + | ||
11 | + this.on('touchend touchcancel', 'span', this.btnClick.bind(this)); | ||
12 | + } | ||
13 | + | ||
14 | + /** | ||
15 | + * 立即领取按钮 | ||
16 | + */ | ||
17 | + btnClick() { | ||
18 | + this.emit('goReceive'); | ||
19 | + } | ||
20 | + | ||
21 | + /** | ||
22 | + * 弹框消失 | ||
23 | + */ | ||
24 | + popHide() { | ||
25 | + setTimeout(function() { | ||
26 | + if ($('.pop-up').css('display') === 'block') { | ||
27 | + $('.pop-up').hide(); | ||
28 | + } | ||
29 | + }, 2000); | ||
30 | + } | ||
31 | +} | ||
32 | + | ||
33 | +module.exports = { | ||
34 | + IndexView | ||
35 | +}; |
1 | +'use strict'; | ||
2 | +const tip = require('plugin/tip'), | ||
3 | + yoho = require('yoho-app'); | ||
4 | + | ||
5 | +import { | ||
6 | + Controller | ||
7 | +} from 'yoho-mvc'; | ||
8 | + | ||
9 | +import { | ||
10 | + ListView | ||
11 | +} from './view'; | ||
12 | + | ||
13 | +import { | ||
14 | + FreeMailList | ||
15 | +} from './model'; | ||
16 | + | ||
17 | +class FreeMailIndexController extends Controller { | ||
18 | + constructor() { | ||
19 | + super(); | ||
20 | + | ||
21 | + this.listView = new ListView(); | ||
22 | + this.listMailModel = new FreeMailList(); | ||
23 | + this.listView.on('receiveCoupon', this.receiveCoupon.bind(this)); | ||
24 | + } | ||
25 | + | ||
26 | + receiveCoupon() { | ||
27 | + this.listMailModel.receiveCoupon().then(result => { | ||
28 | + if (result.code === 200) { | ||
29 | + tip.show('领取成功'); | ||
30 | + | ||
31 | + setTimeout(function() { | ||
32 | + if (yoho.isApp) { | ||
33 | + yoho.goH5('http://m.yohobuy.com/home/coupons', JSON.stringify({ | ||
34 | + action: 'go.coupon', | ||
35 | + params: { | ||
36 | + islogin: 'N', | ||
37 | + url: 'http://m.yohobuy.com/home/coupons' | ||
38 | + } | ||
39 | + })); | ||
40 | + } else { | ||
41 | + location.href = '//m.yohobuy.com/home/coupons'; | ||
42 | + } | ||
43 | + }, 2000); | ||
44 | + } else { | ||
45 | + tip.show(result.message); | ||
46 | + } | ||
47 | + }); | ||
48 | + } | ||
49 | +} | ||
50 | + | ||
51 | +module.exports = FreeMailIndexController; |
public/js/activity/free-mail-list/index.js
0 → 100644
public/js/activity/free-mail-list/model.js
0 → 100644
1 | +'use strict'; | ||
2 | + | ||
3 | +import { | ||
4 | + http | ||
5 | +} from 'yoho-mvc'; | ||
6 | + | ||
7 | +class FreeMailList { | ||
8 | + /** | ||
9 | + * 列表页领取劵 | ||
10 | + */ | ||
11 | + receiveCoupon() { | ||
12 | + return http({ | ||
13 | + type: 'GET', | ||
14 | + url: '/activity/free-mail/verifyCoupon' | ||
15 | + }); | ||
16 | + } | ||
17 | +} | ||
18 | + | ||
19 | +module.exports = { | ||
20 | + FreeMailList | ||
21 | +}; |
public/js/activity/free-mail-list/view.js
0 → 100644
1 | +import { | ||
2 | + View | ||
3 | +} from 'yoho-mvc'; | ||
4 | + | ||
5 | +class ListView extends View { | ||
6 | + constructor() { | ||
7 | + super('.free-mail-list-page'); | ||
8 | + | ||
9 | + this.receiveBtn = $('.receive-btn'); | ||
10 | + | ||
11 | + this.on('touchend touchcancel', '.receive-btn', this.receiveClick.bind(this)); | ||
12 | + } | ||
13 | + | ||
14 | + /** | ||
15 | + * 领券按钮 | ||
16 | + */ | ||
17 | + receiveClick() { | ||
18 | + this.emit('receiveCoupon'); | ||
19 | + } | ||
20 | +} | ||
21 | + | ||
22 | +module.exports = { | ||
23 | + ListView | ||
24 | +}; |
1 | +.free-mail-index-page { | ||
2 | + .top-banner { | ||
3 | + width: 100%; | ||
4 | + height: 375px; | ||
5 | + background-image: resolve("activity/free-mail/banner.png"); | ||
6 | + background-size: 100%; | ||
7 | + background-repeat: no-repeat; | ||
8 | + | ||
9 | + .receive-btn { | ||
10 | + width: 300px; | ||
11 | + height: 60px; | ||
12 | + display: block; | ||
13 | + position: relative; | ||
14 | + top: 278px; | ||
15 | + left: 170px; | ||
16 | + } | ||
17 | + } | ||
18 | + | ||
19 | + .instructions { | ||
20 | + background: #c70e0e; | ||
21 | + width: 100%; | ||
22 | + padding: 30px 25px 40px; | ||
23 | + font-family: "SourceHanSansCN"; | ||
24 | + | ||
25 | + .instructions-detail { | ||
26 | + width: 100%; | ||
27 | + height: 100%; | ||
28 | + background: #fff; | ||
29 | + border-radius: 15px; | ||
30 | + padding: 32px 45px 0; | ||
31 | + } | ||
32 | + | ||
33 | + .title { | ||
34 | + width: 230px; | ||
35 | + height: 51px; | ||
36 | + background-image: resolve("activity/free-mail/title.png"); | ||
37 | + background-size: 100%; | ||
38 | + background-repeat: no-repeat; | ||
39 | + margin: 0 133px 35px; | ||
40 | + } | ||
41 | + | ||
42 | + .color { | ||
43 | + color: #c70e0e; | ||
44 | + } | ||
45 | + } | ||
46 | + | ||
47 | + .detail-item { | ||
48 | + width: 100%; | ||
49 | + margin-bottom: 30px; | ||
50 | + font-weight: 700; | ||
51 | + position: relative; | ||
52 | + | ||
53 | + .item-left { | ||
54 | + width: 82px; | ||
55 | + margin-right: 15px; | ||
56 | + position: absolute; | ||
57 | + top: 50%; | ||
58 | + transform: translate(-50%, -50%); | ||
59 | + margin-left: 20px; | ||
60 | + | ||
61 | + .item-title { | ||
62 | + font-size: 15px; | ||
63 | + font-weight: 700; | ||
64 | + text-align: center; | ||
65 | + } | ||
66 | + } | ||
67 | + | ||
68 | + .item-right { | ||
69 | + font-size: 20px; | ||
70 | + line-height: 36px; | ||
71 | + margin-left: 85px; | ||
72 | + | ||
73 | + a { | ||
74 | + border-bottom: 2px solid #c70e0e; | ||
75 | + } | ||
76 | + } | ||
77 | + | ||
78 | + .icon { | ||
79 | + display: block; | ||
80 | + width: 67px; | ||
81 | + height: 45px; | ||
82 | + margin-left: 7px; | ||
83 | + } | ||
84 | + } | ||
85 | + | ||
86 | + .time { | ||
87 | + .icon { | ||
88 | + background-image: resolve("activity/free-mail/time.png"); | ||
89 | + background-size: 100%; | ||
90 | + background-repeat: no-repeat; | ||
91 | + } | ||
92 | + } | ||
93 | + | ||
94 | + .status { | ||
95 | + .icon { | ||
96 | + background-image: resolve("activity/free-mail/status.png"); | ||
97 | + background-size: 100% 98%; | ||
98 | + background-repeat: no-repeat; | ||
99 | + } | ||
100 | + } | ||
101 | + | ||
102 | + .tip { | ||
103 | + .icon { | ||
104 | + background-image: resolve("activity/free-mail/tip.png"); | ||
105 | + background-size: 100% 98%; | ||
106 | + background-repeat: no-repeat; | ||
107 | + } | ||
108 | + } | ||
109 | + | ||
110 | + .pop-up { | ||
111 | + position: fixed; | ||
112 | + text-align: center; | ||
113 | + width: 405px; | ||
114 | + padding: 15PX; | ||
115 | + top: 500px; | ||
116 | + left: 50%; | ||
117 | + margin-left: -30%; | ||
118 | + margin-top: -170px; | ||
119 | + background-color: #959595; | ||
120 | + color: #fff; | ||
121 | + font-size: 18PX; | ||
122 | + border: none; | ||
123 | + z-index: 100; | ||
124 | + box-sizing: border-box; | ||
125 | + border-radius: 42px; | ||
126 | + display: none; | ||
127 | + | ||
128 | + .details { | ||
129 | + padding-top: 5px; | ||
130 | + | ||
131 | + span { | ||
132 | + display: block; | ||
133 | + width: 91px; | ||
134 | + height: 91px; | ||
135 | + background-image: resolve("activity/free-mail/failure.png"); | ||
136 | + background-repeat: no-repeat; | ||
137 | + background-size: 100%; | ||
138 | + position: relative; | ||
139 | + left: 50%; | ||
140 | + margin-left: -46px; | ||
141 | + margin-bottom: 14px; | ||
142 | + } | ||
143 | + } | ||
144 | + } | ||
145 | + | ||
146 | + .big { | ||
147 | + font-size: 36px; | ||
148 | + padding: 30px 0; | ||
149 | + } | ||
150 | +} |
public/scss/activity/free-mail-list.page.css
0 → 100644
1 | +.free-mail-list-page { | ||
2 | + .top-banner { | ||
3 | + width: 100%; | ||
4 | + height: 245px; | ||
5 | + background-image: resolve("activity/free-mail/list-banner.png"); | ||
6 | + background-repeat: no-repeat; | ||
7 | + background-size: 100%; | ||
8 | + margin-bottom: 15px; | ||
9 | + | ||
10 | + .coupon-name { | ||
11 | + display: inline-block; | ||
12 | + font-size: 45px; | ||
13 | + color: #fff; | ||
14 | + font-family: "SourceHanSansCN"; | ||
15 | + transform: rotate(-9.5deg); | ||
16 | + margin: 40px 0 0 186px; | ||
17 | + font-weight: 700; | ||
18 | + } | ||
19 | + } | ||
20 | + | ||
21 | + .coupon-list { | ||
22 | + padding: 0 35px; | ||
23 | + color: #fff; | ||
24 | + max-height: 452px; | ||
25 | + overflow-y: scroll; | ||
26 | + | ||
27 | + .coupon-item { | ||
28 | + width: 570px; | ||
29 | + height: 135px; | ||
30 | + background-image: resolve("activity/free-mail/coupon.png"); | ||
31 | + background-size: 100%; | ||
32 | + background-repeat: no-repeat; | ||
33 | + margin-bottom: 10px; | ||
34 | + } | ||
35 | + | ||
36 | + .item-left { | ||
37 | + padding-left: 110px; | ||
38 | + font-family: "SourceHanSansCN"; | ||
39 | + width: 340px; | ||
40 | + height: 100%; | ||
41 | + display: inline-block; | ||
42 | + } | ||
43 | + | ||
44 | + .name { | ||
45 | + font-size: 42px; | ||
46 | + font-weight: 700; | ||
47 | + display: inline-block; | ||
48 | + max-width: 205px; | ||
49 | + overflow: hidden; | ||
50 | + white-space: nowrap; | ||
51 | + text-overflow: ellipsis; | ||
52 | + } | ||
53 | + | ||
54 | + .time { | ||
55 | + font-size: 12px; | ||
56 | + } | ||
57 | + | ||
58 | + .item-right { | ||
59 | + height: 100%; | ||
60 | + display: inline-block; | ||
61 | + font-size: 101px; | ||
62 | + font-family: "IKEASans"; | ||
63 | + padding-left: 74px; | ||
64 | + font-weight: 800; | ||
65 | + } | ||
66 | + } | ||
67 | + | ||
68 | + .receive-btn { | ||
69 | + height: 116px; | ||
70 | + width: 100%; | ||
71 | + background-image: resolve("activity/free-mail/list-btn.png"); | ||
72 | + background-size: 100%; | ||
73 | + background-repeat: no-repeat; | ||
74 | + } | ||
75 | +} |
-
Please register or login to post a comment