Merge branch 'feature/freeMailAct' into 'release/6.0'
免邮券领取页 See merge request !740
Showing
24 changed files
with
595 additions
and
0 deletions
apps/activity/controllers/free-mail.js
0 → 100644
apps/activity/models/free-mail.js
0 → 100644
public/img/activity/free-mail/banner.png
0 → 100644

229 KB
public/img/activity/free-mail/coupon.png
0 → 100644

12.9 KB
public/img/activity/free-mail/failure.png
0 → 100644

6.1 KB

153 KB
public/img/activity/free-mail/list-btn.png
0 → 100644

17.2 KB
public/img/activity/free-mail/status.png
0 → 100644

4.24 KB
public/img/activity/free-mail/time.png
0 → 100644

4.45 KB
public/img/activity/free-mail/tip.png
0 → 100644

3.91 KB
public/img/activity/free-mail/title.png
0 → 100644
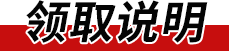
8.88 KB
public/js/activity/free-mail-index/index.js
0 → 100644
public/js/activity/free-mail-index/model.js
0 → 100644
public/js/activity/free-mail-index/view.js
0 → 100644
public/js/activity/free-mail-list/index.js
0 → 100644
public/js/activity/free-mail-list/model.js
0 → 100644
public/js/activity/free-mail-list/view.js
0 → 100644
public/scss/activity/free-mail-list.page.css
0 → 100644
-
Please register or login to post a comment