Merge branch 'feature/index' into develop
Showing
39 changed files
with
4323 additions
and
55 deletions
apps/channel/views/partials/commodity.hbs
0 → 100644
apps/channel/views/partials/floor-header.hbs
0 → 100644
doraemon/views/partial/banner.hbs
0 → 100644
doraemon/views/partial/floor-header.hbs
0 → 100644
public/img/index/brands-layer.png
0 → 100644
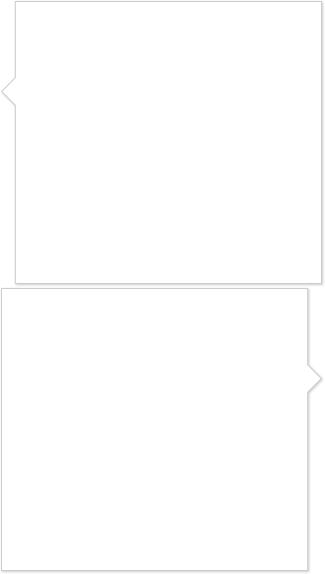
2.43 KB
public/img/index/logo-brand-line.png
0 → 100644

2.27 KB
public/img/index/mars.png
0 → 100644

17.8 KB
public/img/index/play.png
0 → 100644

1.55 KB
public/img/index/qr-app.png
0 → 100644

30.2 KB
public/img/index/qr-weibo.png
0 → 100644

328 KB
public/img/index/qr-weixin.png
0 → 100644

201 KB
public/img/index/show.png
0 → 100644

26.6 KB
public/img/index/yoho.png
0 → 100644

11.4 KB
public/js/channel/boys.page.js
0 → 100644
public/js/plugins/accordion.js
0 → 100644
public/js/plugins/dialog.js
0 → 100644
public/js/plugins/logo-brand.js
0 → 100644
public/js/plugins/new-arrivls.js
0 → 100644
public/js/plugins/slider.js
0 → 100644
public/js/plugins/slider2.js
0 → 100644
public/js/plugins/yohoui/YH.base.js
0 → 100644
public/js/plugins/yohoui/YH.slide.js
0 → 100644
public/scss/index/_brand.css
0 → 100644
public/scss/index/_coupon.css
0 → 100644
public/scss/index/_index-pliffy.css
0 → 100644
public/scss/index/_index.css
0 → 100644
public/scss/index/_min-index.css
0 → 100644
-
Please register or login to post a comment